How to crop image using ASP.Net/C# OR Cropping image in C# before upload
DOWNLOAD SOURCE CODE FOR CROP IMAGE IN ASP.NET
Crop images before upload to the server in C#/ASP.Net
In our previous post we demonstrate how we can re size image using C# or Create thumbnail image using ASP.Net. Here we are demonstrating how we can crop images using ASP.Net application using Jquery and C#. In some applications we need to upload images and we need only some portion of the images to get clear picture on the photo. In this case we need to give an option to users to crop image before they are uploading the image.
Include Jquery file/CSS files to the application.
First of all we need to include following jquery/CSS files to the application.
1. jquery.min.js – You can download from here
2. jquery.Jcrop.js – You can download from here
3. jquery.Jcrop.css – You can download from here
Simple steps to crop image using ASP.Net,C#,Jquery
In this application we are having a browse option for selecting an image. Once we selected a image it will display int the screen.

Select an image to crop using ASP.Net C# Jquery
In the next step we will have the option to select an area to crop the image. Once we selected an area we can click crop button on the screen.
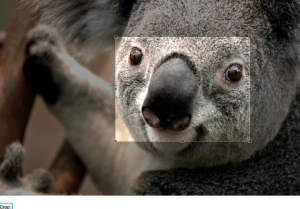
Select image area to crop
Then the selected area will be cropped and displayed in the screen.
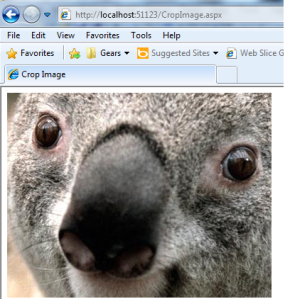
Cropped area of the image
ASPX Page for crop images using Jquery
<%@ Page Language="C#" AutoEventWireup="true" CodeBehind="CropImage.aspx.cs" Inherits="ExperimentLab.CropImage" %> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head id="Head1" runat="server"> <title>Crop Image</title> <link href="Styles/jquery.Jcrop.css" rel="stylesheet" type="text/css" /> <script type="text/javascript" src="http://ajax.googleapis.com/ajax/libs/jquery/1.3/jquery.min.js"></script> <script type="text/javascript" src="Scripts/jquery.Jcrop.js"></script> <script type="text/javascript"> jQuery(document).ready(function () { jQuery('#imgCrop').Jcrop({ onSelect: storeCoords }); }); function storeCoords(c) { jQuery('#X').val(c.x); jQuery('#Y').val(c.y); jQuery('#W').val(c.w); jQuery('#H').val(c.h); }; </script> </head> <body> <form id="form1" runat="server"> <div> <asp:Panel ID="pnlUpload" runat="server"> <asp:FileUpload ID="Upload" runat="server" /> <br /> <asp:Button ID="btnUpload" runat="server" OnClick="btnUpload_Click" Text="Upload" /> <asp:Label ID="lblError" runat="server" Visible="false" /> </asp:Panel> <asp:Panel ID="pnlCrop" runat="server" Visible="false"> <asp:Image ID="imgCrop" runat="server" /> <br /> <asp:HiddenField ID="X" runat="server" /> <asp:HiddenField ID="Y" runat="server" /> <asp:HiddenField ID="W" runat="server" /> <asp:HiddenField ID="H" runat="server" /> <asp:Button ID="btnCrop" runat="server" Text="Crop" OnClick="btnCrop_Click" /> </asp:Panel> <asp:Panel ID="pnlCropped" runat="server" Visible="false"> <asp:Image ID="imgCropped" runat="server" /> </asp:Panel> </div> </form> </body> </html>
Code Behind of the ASPX page for Crop image using ASP.Net/C#
using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.UI; using System.Web.UI.WebControls; using System.IO; using SD = System.Drawing; using System.Drawing.Drawing2D; namespace ExperimentLab { public partial class CropImage : System.Web.UI.Page { protected void Page_Load(object sender, EventArgs e) { } String path = HttpContext.Current.Request.PhysicalApplicationPath + "images\\"; protected void btnUpload_Click(object sender, EventArgs e) { Boolean FileOK = false; Boolean FileSaved = false; if (Upload.HasFile) { Session["WorkingImage"] = Upload.FileName; String FileExtension = Path.GetExtension(Session["WorkingImage"].ToString()).ToLower(); String[] allowedExtensions = { ".png", ".jpeg", ".jpg", ".gif" }; for (int i = 0; i < allowedExtensions.Length; i++) { if (FileExtension == allowedExtensions[i]) { FileOK = true; } } } if (FileOK) { try { Upload.PostedFile.SaveAs(path + Session["WorkingImage"]); FileSaved = true; } catch (Exception ex) { lblError.Text = "File could not be uploaded." + ex.Message.ToString(); lblError.Visible = true; FileSaved = false; } } else { lblError.Text = "Cannot accept files of this type."; lblError.Visible = true; } if (FileSaved) { pnlUpload.Visible = false; pnlCrop.Visible = true; imgCrop.ImageUrl = "images/" + Session["WorkingImage"].ToString(); } } protected void btnCrop_Click(object sender, EventArgs e) { string ImageName = Session["WorkingImage"].ToString(); int w = Convert.ToInt32(W.Value); int h = Convert.ToInt32(H.Value); int x = Convert.ToInt32(X.Value); int y = Convert.ToInt32(Y.Value); byte[] CropImage = Crop(path + ImageName, w, h, x, y); using (MemoryStream ms = new MemoryStream(CropImage, 0, CropImage.Length)) { ms.Write(CropImage, 0, CropImage.Length); using (SD.Image CroppedImage = SD.Image.FromStream(ms, true)) { string SaveTo = path + "crop" + ImageName; CroppedImage.Save(SaveTo, CroppedImage.RawFormat); pnlCrop.Visible = false; pnlCropped.Visible = true; imgCropped.ImageUrl = "images/crop" + ImageName; } } } static byte[] Crop(string Img, int Width, int Height, int X, int Y) { try { using (SD.Image OriginalImage = SD.Image.FromFile(Img)) { using (SD.Bitmap bmp = new SD.Bitmap(Width, Height)) { bmp.SetResolution(OriginalImage.HorizontalResolution, OriginalImage.VerticalResolution); using (SD.Graphics Graphic = SD.Graphics.FromImage(bmp)) { Graphic.SmoothingMode = SmoothingMode.AntiAlias; Graphic.InterpolationMode = InterpolationMode.HighQualityBicubic; Graphic.PixelOffsetMode = PixelOffsetMode.HighQuality; Graphic.DrawImage(OriginalImage, new SD.Rectangle(0, 0, Width, Height), X, Y, Width, Height, SD.GraphicsUnit.Pixel); MemoryStream ms = new MemoryStream(); bmp.Save(ms, OriginalImage.RawFormat); return ms.GetBuffer(); } } } } catch (Exception Ex) { throw (Ex); } } } } DOWNLOAD SOURCE CODE FOR CROP IMAGE IN ASP.NET
Good one.. Is it possible to paste the cropped image where ever required on the form
Sure. You can put img tag wherever you need to display image. Then u can give the source as crpped image
what is ExperimentLab.CropImage in inherits=” ” . it give error.
Hi Satyakrshr,
“ExperimentLab” is namesapce name in the above example. You have to replace this name with your namespace name. Please try and let me know if you have any other issues.
where i found jquery files
Is this possible to do in ASP MVC?
Hi Nikos,
Thank you for visiting my blog.
I didnt try with MVC yet and I will check and let you know.
From where i can get these jquery files….
1. jquery.min.js
2. jquery.Jcrop.js
3. jquery.Jcrop.css
Hi fazal, Thank you for visting my blog. I will upload jquery files and will post the links today evening.
got the jquery files by googling…but i’m unable to select the area for cropping after uploading the image…plz reply
Hi fazal,
Can u share the error that you got. Please check any script error tracked in the browser also.
Thanx tuvian…..M not getting any error….File is getting upload and after getting the saved image on my page m unable to select the area for cropping….
Hi fazal,
Please make sure that u copied entire section of codes in the post. Especially the lines that we included jquery files. Also check the path of the jquery file that u copoed and mapped is correct. Also verify no popup blocking or any other message is not coming from browser. Please try some other browsers also to track the issue.
Hi tuvian,
I tried for mozilla n chrome getting same issue….I think the problem is with jquery files which m using might not b the one U specified….It would be helpfull if u send me the jquery files now instead in evening….
Thanx n regard
Fazal
abu.fazal@magwebonline.com
Awesome looking fwd to MVC code, I’ll end up doing it myself anyway next week!
Hi Fazal,
I Included links for downloading css and js files.
Please download and try again.
Hi Nikos,
I didnt start with MVC yet. If you come across the solution first, please feel to share the same.
I have the uploaded image . Can I just crop the image using the crop code
Hi raghava,
Sure, You can crop the uploaded image using codes mentioned in the post.
Please fee free to ask any queries.
Thanks for visiting our blog
Your post is very good in upload and crop point of view. But I need to save the path of the image in database.I have the code for that and i am in need of the crop code.So can you please help me in this aspect.
Hi Raghava,
You can use above codes for crop images. In your scenario you need to show uploaded image in asp image tag and then crop from there as mentioned in above post.
dear sir your code is not running properly .It shows exception at runtime the error is
Input string was not in a correct format. at crop event.please reply soon
Hi shaliesh,
The above code is working fine in my machine.
Please send your email id so that I will send source code to you.
Hello,
how I replace Experiment.lab namespace…
I m totally new in asp.net
Hi Arnav,
You can replace ExperimentLab with your namespace name. As you are new to ASP.Net, you just copy after the namespace code from above post.
Please try and let me know if you face any problem.
Thank you for visiting blog.. 🙂
Hi All,
I posted a sample application with full source code in the above post.
You can download and run application.
thanks,
Hi
You have given an image control and you are uploading image to that control.
If i Set width and height to that control cropping is not done properly.If i crop in center it is coming from left side.So finally i set height and width to image control cropping is not working properly.Please give me one solution.
Hi Deepthi,
I just tried your scenario and it is working fine here.
1. Just download sample application from above post
2. Open CropImage.aspx file and add Width and height attribute to the asp:Image ID=”imgCrop” (Width=”100″ Height=”100″)
3. Run the application and able to crop the image.
Just try it and let me know any issues you still face.
thanks,
this code worked with mvc
whether this code worked with MVC?
awesome sample – any easy way to only allow a 2×3 ratio for selection? Haven’t dug into the plugin yet.
Hi Tuvian.,
Thanks for giving Awesome code i need code for saved the image to DB after Cropping was done Give any suggestions ASAP..
Thks,
Suman.
Can any one Give a suggestions for imaged saved to db after cropping done.
After press submit button the image saved to Db table.
Thks,
Suman.
Hi Suman,
One way to achieve the requirement is, upload the cropped image into the server.
Then save the URL of the uploaded image into the db as string.
Thanks
Hi Tuvian Thanks for your suggestion I have one more Question After cropping was done the image displays in same window or new window it will be again cropped to depend upon user compatibility how to add that functionality to this Example plz give me sample code…
Thanks
I hope this article has given you some useful facts
about roofs. Nash, who had received a gigantic claim in reward for his discovery,
could possibly be seen nearly every day busily at work.
As the atmosphere began to disintegrate around me, I felt a fresh
strength forge into my thoughts.
asfadf
For example, being looking for film, it is possible to select files
by limiting their size – applying this option you are going to except
low-quality films (SAT-Rip, CAM-Rip, Telesync).
As daylight broke from a miserable night a helicopter flew in, dropping a line to
tug the man to safety. When Vista Service Pack 1 was within the beta phase, Microsoft included a little software utility called ‘recdisc.
If you try to create your individual torrent then a instructions on how to use torrents and how to
make torrents are usually in the torrent program you have installed on
your computer. Whether you use it in your own home, at college or at
the office Ubuntu contains each of the applications
you’ll ever need, from word processing and email applications,
to web server software and programming tools. When Vista Service Pack 1 was inside
the beta phase, Microsoft included a little software utility called ‘recdisc.
But during the last few years, IPTV has dwarfed the grade of DVD and
Cable TV. I am not really a PC player, please anyone
let me to remove Dell Inspiron password…” We usually hear such inquiry around us.
We’ll start our tour at Marina Grande for the north shore from the
island, about one third with the way in looking at the easternmost point.